Let's discuss C# books. When learning the language, sometimes it helps to have reference material handy. That's especially true for this language. Programmers of all skill levels rely on C# books.
The Best C# Books to Learn the Language
So you've decided to dive into the cool world of C# programming. Whether you're just starting out or you've been coding for ages, you know that a good book can be a game-changer. C# has been around since 2000, and boy, has it seen some changes!
From the basics to all the snazzy stuff like LINQ and async programming, there's a lot to cover. But, hey, don't get lost in the techy maze! We've sifted through stacks of books to bring you the best of the best.
This comprehensive book review article can also help you earn a C# certification. Ready to get started?
Grab a cup of coffee, pull up a chair, and let's explore the top C# books that'll make your coding journey a breeze.
1. C# 10 and .NET 6 - Modern Cross-Platform Development
This C# book is like the cozy beginner's guide to the programming party. The author dives into all the must-know basics and even teases some of the fancy advanced stuff. Instead of just telling you what to do, they spill the beans on why it works that way in C#.
The best part? The straightforward style and a bucket-load of examples for every topic. Two thumbs up for keeping it real and easy to follow!
Overall, this book is a great start for learning C#, whether you are a new programmer or an experienced one. Plus, it's the sixth edition from Mark J. Price. That's the latest version available!
Features
-
It starts with basics like installing Visual Studio and Visual Studio and advanced topics like performance tuning and data manipulations using Entity Framework and LINQ.
-
Includes lots of information on C# 10, the .NET 6 class library, the latest available
-
Includes bonus chapters on building mobile and desktop apps
-
Structured approach and a well-organized flow of topics
-
Features fundamentals for the C# programming language, but assumes you already have a programming background
2. Programming C# 8.0 (O’Reilly)
If you're kinda past the newbie stage and want to step up your C# game, this O’Reilly book is like your trusty sidekick. The author's got this knack for pulling out relatable examples that'll stick in your brain. It's packed, detailed, and gives you the lowdown on every nook and cranny of C#.
Quick heads-up: If you're looking for stuff on Visual Studio or the .NET framework, you might wanna look elsewhere. This book kinda assumes you've danced with at least one programming language before (doesn't have to be C#, any old tango partner will do).
Dreaming of building a killer web app or designing the next big Unity game? Then, buddy, this book's calling your name!
Features
-
The author’s style is fluent and crisp, showing his experience and thorough understanding of C#.
-
Advanced topics like garbage collection, exceptions, delegates, and events are explained well.
-
If you are new to programming, it is advisable first to read the older version of the book, which has more basics than this book (like object oriented programming concepts, the basic syntax of C#, etc.)
-
The author gives a lot of tips, notes, and suggestions that enhance the reader’s knowledge.
-
The book follows a guided approach, and you can look up the web for topics you want to know more about with the link given or the topic name.
-
Note that there's a kindle version available too!
3. Murach's C# 2015 6th Edition
Alright, listen up, code enthusiasts! This book? Yeah, it's the 6th Edition, but man, it's like that vintage record you can't help but love. Whether you're just strapping on your C# boots or you've been trekking in the coding wilderness for years, this book's got your back.
No long-winded chats here – the author gets straight to the point and serves up some neat code examples that flow smoother than your morning coffee.
Now, if you're already BFFs with Visual Studio, you might wanna zoom past the starter chapters. And here's a fun twist: instead of the usual console examples, this bad boy's all about Windows forms – something the author's pretty stoked about.
Grab it, read it, and add it to your tech treasure trove!
Features
-
The book provides practical examples that you can relate to when you are doing real-time projects.
-
The content is clear, concise, and easy to understand.
-
The author has a great teaching style, and the book is well-organized.
-
A good guide for reference as well as self-study.
-
Covers all the basic OOP concepts as well as builds professional-level applications with much more complex concepts.
-
The author gives a lot of useful tips and explains concepts through pictures throughout the chapters.
4. The C# Player's Guide (3rd Edition) 3rd Edition
Alright, all you budding C# rockstars, here's the deal: if you're just dipping your toes into the vast ocean of coding, this book's like your lifeboat. It kicks off with the ABCs of programming.
So, even if you've never written a line of code before, don't sweat it – it's got your beginner's vibes covered, especially with some in-depth chats about OOP.
Now, a tiny hint: if you've dabbled in C/C++ (shout out to all the engineering students!), you've got a bit of a head start. But either way, the book's a treat. Think of it like a six-course meal – the first half gives you those delicious basics, while the latter three parts turn up the heat with some intermediate goodness. Dig in!
Features
-
Focuses on learning C# rather than introducing the reader to multiple tools.
-
Covers all the OOP concepts, including generics, in detail.
-
A lot of exercises and challenges at the end of each chapter contain the right amount of difficulty.
-
The book has some bits that are not fully explored, so it is good to supplement those topics with online blogs for more information.
-
Acts as a good reference for those who want to brush up their C# knowledge.
5. C# in Depth: Fourth Edition 4th Edition
You know how we all end up on StackOverflow during those late-night coding sessions? Well, guess what? One of their top contributors went and penned this book. Yeah, it's the 4th edition, but don’t let that number fool ya – it dives deep into the cool bits of C# 6 & 7.
This ain't just your typical how-to guide. Oh no! It takes you on a brainy journey, making you "get" C# in a way that's both clear and kinda fun.
It's like the author's in your head, answering the 'how' and the 'why' before you even ask. Quick note: if you're past the beginner's playground and diving into the deeper end of coding, this book's got your name on it!
Features
-
Since a community contributor writes it, the approach followed is efficient, keeping in mind the common problems developers face.
-
This is not the ideal book if you have no idea what C# is, but a great one if you knowC#e basics like data types, important C# features, and writing simple code.
-
The book covers just the right amount of information for intermediate learners – not too detailed and not too high-level.
-
The author spends a great amount of time explaining the new features and C# evolution over the years.
6. Microsoft Visual C# Step by Step (Developer Reference) 8th Edition
If you've got some downtime before your next big project kicks off, this book's gonna be your new BFF. Think of it as laying down a rock-solid foundation for all those epic coding adventures coming your way. The author dives deep, like scuba-diving-in-the-C#-ocean deep, explaining all those juicy core concepts and the stories behind them.
One cool thing? Aside from some killer code examples, the author's gone and added diagrams – a sweet little touch that makes stuff so much clearer. (Kinda wish more books did that!)
Whether you're fresh off the coding boat or somewhere in the middle, this book's got your back. New to the game? The author’s got a roadmap just for you – cover to cover.
But if you've already grooved with C, just skim through the early chapters and jump into the good stuff. Enjoy the ride!
Features
-
Clear, detailed, and concise – no verbosity.
-
The exercises need Windows 10 to run.
-
Covers both Visual Studio and C# in detail.
-
If this is your first-ever book, you should also supplement your learning with some online material, especially on OOP concepts, as the author brushes through those in the beginning (however, covers them in later chapters).
-
The author gives lots of notes which provide insights on possible alternative approaches for solving a problem.
-
The book is well-organized, and it is easy to navigate and find information quickly.
7. Pro C# 7: With .NET and .NET Core 8th ed. Edition
So, here's the skinny: if you're already past those beginner hurdles and you're looking for something a tad spicier, this book's got your name written all over it. It's got pace, and it ain't holding back! If you're a total newbie, you might wanna start somewhere else. But if you've got a bit of C# (or even C) under your belt, dive on in.
Think of this book as that trusty sidekick you need when climbing the C# career ladder. And the cherry on top? It's not just about what's on the pages.
The author gives you a nudge, encouraging you to dive deeper online and really sink your teeth into some concepts. So, if you're all about leveling up your C# game, grab this gem and get reading!
Features
-
It can be used as a quick reference or a study guide.
-
Thorough explanation of examples (a lot of examples).
-
If you are planning for C# certification, this is your go-to book.
-
All in one book also covers .NET Core, .NET API, Entity Framework, WPF, etc.
-
Exhaustive and dense, take time to read and absorb the concepts.
9. C# 9.0 in a Nutshell: The Definitive Reference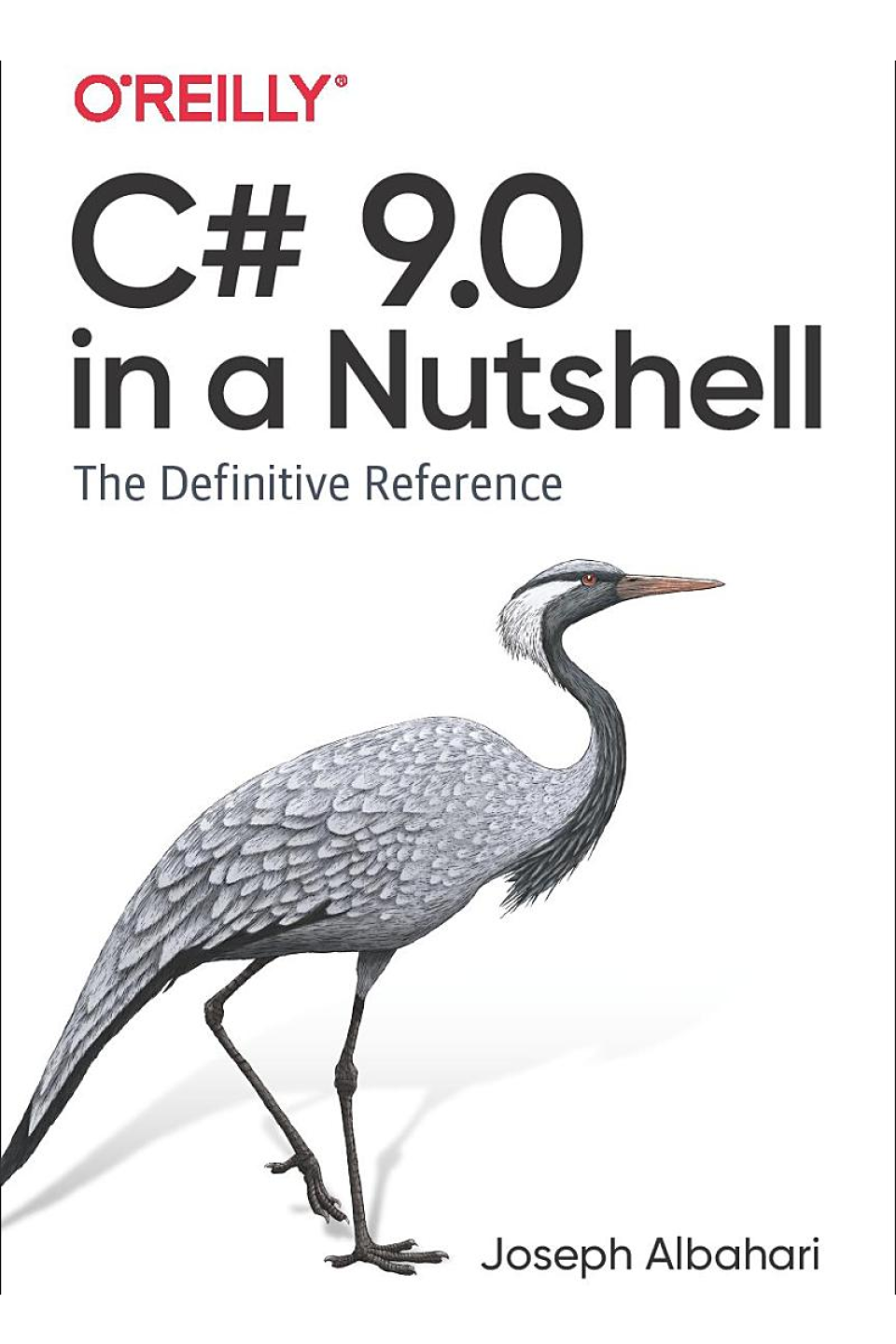
If you're past those beginner blues and hungry for some meatier stuff, this book's the ticket. It dives deep into the complex depths of C#, but don't fret - the author's got style. It starts you off with a bird's-eye view and then, chapter by chapter, takes you on a deeper dive.
What's cool about this book? It strikes that sweet balance between brainy concepts and hands-on practice through real-world examples.
And hey, even if you're just getting your feet wet in programming, as long as you've got those basics down, this book's got a seat saved just for you. Dive in and soak up the knowledge!
Features
-
Clear, concise, without any verbosity, every line is full of meaning and knowledge.
-
Take time to read – it's purely technical and a great reference.
-
Three chapters dedicated to LINQ
-
Includes coverage of .NET features, including cryptography
9. Head First C#: A Learner's Guide to Real-World Programming with C# and .NET Core
Headfirst books are known for their unique visual way of explaining concepts. The book has just the right amount of technical details and provides a good foundation before reading more advanced books on C#.
The authors' funny, witty, and friendly tone compensates for the verbiage here and there. It quickly gets you on to coding and presents many side bits and information that enhance learning.
You can use the book for learning new concepts or as a way to refresh your grasp on topics you haven't used recently.
Features
-
It is not a thorough or pure technical book and provides enough working knowledge to start writing C# code.
-
It presents an intuitive way of learning and doesn’t throw in a lot of content at once.
-
A great book for beginners, where the author focuses on building a strong foundation for the concepts.
-
The author mentions some advanced concepts at a very high level but doesn’t get into too many details.
-
The author explains why something would work and why something will NOT work, giving you an overall understanding of code and concepts.
Bonus C# books
So, those 10 books we chatted about? Absolute gold for leveling up your C# skills at every stage of your journey. But wait, there's more! For all you gaming buffs out there, we've got a bonus round: two rad books that mix C# with a dash of gaming magic.
Heard of Unity? (Of course, you have! We wrote a similar article evaluating Unity books.) It's like the rockstar of gaming engines.
These cherry-picked books are all about mastering C# and Unity together. Game on and happy coding!
1. Learning C# by Developing Games with Unity
The best part of this book is that it starts with the most basic concepts and then covers the most advanced topics! You start by writing small bits of code and develop your skills throughout the book to build more complex systems with the Unity interface.
It may be a bit confusing and fast for beginners at first, but take it slow, and you will be able to follow it. You can supplement your learning with online reference materials.
Features
-
A lot of code examples with thorough explanations.
-
The book is divided into different sections, the first section covers C# in detail, the second covers Unity, and the last section introduces you to more advanced concepts.
-
There are a lot of quizzes at the end of each chapter covered in the book.
-
The author covers various challenges and problems faced during practical game design, giving you a feel of a real-time gaming design experience.
2. Learning C# Programming with Unity 3D, second edition
If you have no prior gaming experience, this is the right book to start. The author introduces readers to a lot of example codes and experiments to build a strong foundation. Even basic code and common programming tasks are focused on gaming and presented from a gaming point of view. This is one book where you can truly appreciate the power of C# as you will see it in action.
Features
-
An interactive and practical approach to game design.
-
Thorough explanation of Unity and C# and how both are used together.
-
The author introduces you to a lot of tips and tricks in the world of gaming.
-
The book also touches upon Git and its use, although you can skip it if you wish.
What is C#?
When we talk about C#, the first thing that comes to mind is ‘speed.’ This is the main reason why C# is used for gaming, VR, mobile apps, desktop applications, web applications, websites, and more. C# is easy to learn and, thus, the most popular programming language in the world. It also has a huge vibrant community.
Books are a great way to learn C#, and an IDE goes hand in hand with practice. Applications written in C# use the .NET framework, so Visual Studio is ideal for IDE on Windows.
C#, pronounced as C sharp, is a general-purpose, multi-paradigm programming language, which is object-oriented. It is a strongly typed language developed by Microsoft in 2000 as part of the .NET initiative. Although C# claims that C# is similar to Java, its design principles are mostly based on C++.
C# is one of the languages for Common Language Infrastructure (CLI). A simple example of a C# program:
using System;
namespace WelcomeToHackr
{
class WelcomeMsg
{
// Main function
static void Main(string[] args)
{
Console.WriteLine("Welcome to Hackr.io");
Console.ReadKey();
}
}
}
This will print ‘Welcome to Hackr.io’ when executed.
Features of C#
C# has many interesting and unique features which make it most suitable for developing games, mobile apps, desktop apps:
-
Automatic garbage collection.
-
No memory leaks, as memory backup is high.
-
Follows OOP concepts (that's Object Oriented Programming, btw) similar to other high-level languages like C, C++, and Java.
-
Structured and component-oriented language.
-
Highly Scalable.
-
Rich libraries, high speed, and performance.
How to Learn C#?
Just like other programming languages, you can easily learn C# by practice! As a starting point, learn the basic concepts and syntax.
If you are new to programming, consider spending some time reading blogs about programming and its basics like data structures, control structures, etc. Hackr.io provides the most popular online C# courses and tutorials.
However, there is nothing like having books with you. With books, you can learn at your own pace. Many nice, informative books teach from scratch and move on to an advanced level.
In the preceding sections, we listed some of the best books of all levels for self-learning, project references, and professional use. This includes basic and more complex topics.
Conclusion
Well, that’s all the information we compiled about the books, friends! Our focus centered on the best books for each level, including intermediate and advanced programmers.
You can start your C# journey with any of the beginner books, like, Murach's C# 2015 6th Edition or The C# Player's Guide (3rd Edition) will be a good starting point. For more experienced learners, starting with Programming C# 8.0 (O’Reilly) and C# in Depth: Fourth Edition 4th Edition will be a good learning curve. Happy reading!
People are also reading: